PyOpenCV 实战:借助视觉识别技术实现围棋终局的胜负判定
前言
学习一种技能,最好的方式就是与实际应用相结合,也就是人们常说的学以致用。很多的 Python 初学者在学完基础语法、基本技能之后,都会感到迷茫,主要原因就是不知道自己的一身本领可以用在何处,接下来又该向哪个方向发展。如果你恰好处在这样一个阶段,又对视觉识别技术感兴趣的话,不妨拿这个项目来练练手。
谈到视觉识别,一定离不开 OpenCV,而 OpenCV 的图像数据结构,就是 NumPy 的多维数组(numpy.ndarray)。在后面的讲解中,我会穿插一些 OpenCV 的基本概念和技术细节,但不会对 NumPy 做过多介绍。
准备工作
2.1 约定围棋局面的数据结构
标准的 19 路围棋盘上有 361 个交叉点可以落子,每个交叉点有三种状态:无子、黑子、白子,不难算出围棋共有 3 的 361 次方种不同的局面。
对于围棋局面,用什么样的数据结构来表示最合理呢?
我以前写过象棋和国际跳棋的代码,对于这两种棋,是用字符串来表示一个局面。考虑到围棋终局时需要统计黑白双方的棋子数量和围空数量,这里选择使用二维的 NumPy 数组来表示围棋局面,数组元素 0 表示无子,1 表示黑子,2 表示白子。下面是我手动输入的一个围棋局面。
import numpy as np
phase = np.array([
[0,0,2,1,1,0,1,1,1,2,0,2,0,2,1,0,1,0,0],
[0,0,2,1,0,1,1,1,2,0,2,0,2,2,1,1,1,0,0],
[0,0,2,1,1,0,0,1,2,2,0,2,0,2,1,0,1,0,0],
[0,2,1,0,1,1,0,1,2,0,2,2,2,0,2,1,0,1,0],
[0,2,1,1,0,1,1,2,2,2,2,0,0,2,2,1,0,1,0],
[0,0,2,1,1,1,1,2,0,2,0,2,0,0,2,1,0,0,0],
[0,0,2,2,2,2,1,2,2,0,0,0,0,0,2,1,0,0,0],
[2,2,2,0,0,0,2,1,1,2,0,2,0,0,2,1,0,0,0],
[1,1,2,0,0,0,2,2,1,2,0,0,0,0,2,1,0,0,0],
[1,0,1,2,0,2,1,1,1,1,2,2,2,0,2,1,1,1,1],
[0,1,1,2,0,2,1,0,0,0,1,2,0,2,2,1,0,0,1],
[1,1,2,2,2,2,2,1,0,0,1,2,2,0,2,1,0,0,0],
[2,2,0,2,2,0,2,1,0,0,1,2,0,2,2,2,1,0,0],
[0,2,0,0,0,0,2,1,0,1,1,2,2,0,2,1,0,0,0],
[0,2,0,0,0,2,1,0,0,1,0,1,1,2,2,1,0,0,0],
[0,0,2,0,2,2,1,1,1,1,0,1,0,1,1,0,0,0,0],
[0,2,2,0,2,1,0,0,0,0,1,0,0,0,0,1,1,0,0],
[0,0,2,0,2,1,0,1,1,0,0,1,0,1,0,1,0,0,0],
[0,0,0,2,1,1,0,0,0,0,0,0,0,0,1,0,0,0,0]
], dtype=np.ubyte)
2.2 显示一个围棋局面
怎样直观地显示一个围棋局面呢?下面的代码使用 Python 的内置函数 print()就可以在控制台上打印出像照片一样的彩色棋盘。
import os
import numpy as np
os.system('')
def show_phase(phase):
"""显示局面"""
for i in range(19):
for j in range(19):
if phase[i,j] == 1:
chessman = chr(0x25cf)
elif phase[i,j] == 2:
chessman = chr(0x25cb)
elif phase[i,j] == 9:
chessman = chr(0x2606)
else:
if i == 0:
if j == 0:
chessman = '%s '%chr(0x250c)
elif j == 18:
chessman = '%s '%chr(0x2510)
else:
chessman = '%s '%chr(0x252c)
elif i == 18:
if j == 0:
chessman = '%s '%chr(0x2514)
elif j == 18:
chessman = '%s '%chr(0x2518)
else:
chessman = '%s '%chr(0x2534)
elif j == 0:
chessman = '%s '%chr(0x251c)
elif j == 18:
chessman = '%s '%chr(0x2524)
else:
chessman = '%s '%chr(0x253c)
print('\033[0;30;43m' + chessman + '\033[0m', end='')
print()
phase = np.array([
[0,0,2,1,1,0,1,1,1,2,0,2,0,2,1,0,1,0,0],
[0,0,2,1,0,1,1,1,2,0,2,0,2,2,1,1,1,0,0],
[0,0,2,1,1,0,0,1,2,2,0,2,0,2,1,0,1,0,0],
[0,2,1,0,1,1,0,1,2,0,2,2,2,0,2,1,0,1,0],
[0,2,1,1,0,1,1,2,2,2,2,0,0,2,2,1,0,1,0],
[0,0,2,1,1,1,1,2,0,2,0,2,0,0,2,1,0,0,0],
[0,0,2,2,2,2,1,2,2,0,0,0,0,0,2,1,0,0,0],
[2,2,2,0,0,0,2,1,1,2,0,2,0,0,2,1,0,0,0],
[1,1,2,0,0,0,2,2,1,2,0,0,0,0,2,1,0,0,0],
[1,0,1,2,0,2,1,1,1,1,2,2,2,0,2,1,1,1,1],
[0,1,1,2,0,2,1,0,0,0,1,2,0,2,2,1,0,0,1],
[1,1,2,2,2,2,2,1,0,0,1,2,2,0,2,1,0,0,0],
[2,2,0,2,2,0,2,1,0,0,1,2,0,2,2,2,1,0,0],
[0,2,0,0,0,0,2,1,0,1,1,2,2,0,2,1,0,0,0],
[0,2,0,0,0,2,1,0,0,1,0,1,1,2,2,1,0,0,0],
[0,0,2,0,2,2,1,1,1,1,0,1,0,1,1,0,0,0,0],
[0,2,2,0,2,1,0,0,0,0,1,0,0,0,0,1,1,0,0],
[0,0,2,0,2,1,0,1,1,0,0,1,0,1,0,1,0,0,0],
[0,0,0,2,1,1,0,0,0,0,0,0,0,0,1,0,0,0,0]
], dtype=np.ubyte)
show_phase(phase)
这段代码的运行结果如下:
2.3 计算黑白双方的棋子和围空
判断一个围棋局面是否为终局,是有一定难度的。为了简化代码,约定提交判决的围棋局面必须是双方死棋、残子均已提清,无任何异议。如此,代码就非常简单了。
import numpy as np
def find_blank(phase, cell):
"""找出包含cell的成片的空格"""
def _find_blank(phase, result, cell):
i, j = cell
phase[i,j] = 9
result['cross'].add(cell)
if i-1 > -1:
if phase[i-1,j] == 0:
_find_blank(phase, result, (i-1,j))
elif phase[i-1,j] == 1:
result['b_around'].add((i-1,j))
elif phase[i-1,j] == 2:
result['w_around'].add((i-1,j))
if i+1 < 19:
if phase[i+1,j] == 0:
_find_blank(phase, result, (i+1,j))
elif phase[i+1,j] == 1:
result['b_around'].add((i+1,j))
elif phase[i+1,j] == 2:
result['w_around'].add((i+1,j))
if j-1 > -1:
if phase[i,j-1] == 0:
_find_blank(phase, result, (i,j-1))
elif phase[i,j-1] == 1:
result['b_around'].add((i,j-1))
elif phase[i,j-1] == 2:
result['w_around'].add((i,j-1))
if j+1 < 19:
if phase[i,j+1] == 0:
_find_blank(phase, result, (i,j+1))
elif phase[i,j+1] == 1:
result['b_around'].add((i,j+1))
elif phase[i,j+1] == 2:
result['w_around'].add((i,j+1))
result = {'cross':set(), 'b_around':set(), 'w_around':set()}
_find_blank(phase, result, cell)
return result
def find_blanks(phase):
"""找出所有成片的空格"""
blanks = list()
while True:
cells = np.where(phase==0)
if cells[0].size == 0:
break
blanks.append(find_blank(phase, (cells[0][0], cells[1][0])))
return blanks
def stats(phase):
"""统计结果"""
temp = np.copy(phase)
for item in find_blanks(np.copy(phase)):
if len(item['w_around']) == 0:
v = 3 # 黑空
elif len(item['b_around']) == 0:
v = 4 # 白空
else:
v = 9 # 单官或公气
for i, j in item['cross']:
temp[i, j] = v
black = temp[temp==1].size + temp[temp==3].size
white = temp[temp==2].size + temp[temp==4].size
common = temp[temp==9].size
return black, white, common
if __name__ == '__main__':
phase = np.array([
[0,0,2,1,1,0,1,1,1,2,0,2,0,2,1,0,1,0,0],
[0,0,2,1,0,1,1,1,2,0,2,0,2,2,1,1,1,0,0],
[0,0,2,1,1,0,0,1,2,2,0,2,0,2,1,0,1,0,0],
[0,2,1,0,1,1,0,1,2,0,2,2,2,0,2,1,0,1,0],
[0,2,1,1,0,1,1,2,2,2,2,0,0,2,2,1,0,1,0],
[0,0,2,1,1,1,1,2,0,2,0,2,0,0,2,1,0,0,0],
[0,0,2,2,2,2,1,2,2,0,0,0,0,0,2,1,0,0,0],
[2,2,2,0,0,0,2,1,1,2,0,2,0,0,2,1,0,0,0],
[1,1,2,0,0,0,2,2,1,2,0,0,0,0,2,1,0,0,0],
[1,0,1,2,0,2,1,1,1,1,2,2,2,0,2,1,1,1,1],
[0,1,1,2,0,2,1,0,0,0,1,2,0,2,2,1,0,0,1],
[1,1,2,2,2,2,2,1,0,0,1,2,2,0,2,1,0,0,0],
[2,2,0,2,2,0,2,1,0,0,1,2,0,2,2,2,1,0,0],
[0,2,0,0,0,0,2,1,0,1,1,2,2,0,2,1,0,0,0],
[0,2,0,0,0,2,1,0,0,1,0,1,1,2,2,1,0,0,0],
[0,0,2,0,2,2,1,1,1,1,0,1,0,1,1,0,0,0,0],
[0,2,2,0,2,1,0,0,0,0,1,0,0,0,0,1,1,0,0],
[0,0,2,0,2,1,0,1,1,0,0,1,0,1,0,1,0,0,0],
[0,0,0,2,1,1,0,0,0,0,0,0,0,0,1,0,0,0,0]
], dtype=np.ubyte)
show_phase(phase)
black, white, common = stats(phase)
print('--------------------------------------')
print('黑方:%d,白方:%d,公气:%d'%(black, white, common))
代码运行结果如下图所示。
这段代码在查找成片的空地时使用了递归的方式,这是解决此类问题的有效手段。
处理流程
为了便于查看各种数据结构、对象的信息,整个处理流程我放在 IDLE 中运行。除了标准库,全部代码仅需另外导入 PyOpenCV 和 NumPy 模块。
>>> import cv2
>>> import numpy as np
3.1 图像预处理
做视觉识别,通常要在读入图像之后对读入的图像做一些预处理。比如,在边缘检测或直线检测、圆检测之前,要将彩色图像转为灰度图像,如有必要,还要进行滤波降噪处理,甚至是侵蚀、膨胀处理。
下图是一幅围棋局面的照片,整个处理过程就用这张照片来演示。这个局面是随便摆出来的,并不是一个终局。
下面的代码使用 opencv 打开这张照片,转为灰度图像,对图像进行滤波降噪处理后做边缘检测。
>>> pic_file = r'D:\temp\go\res\pic_0.jpg'
>>> im_bgr = cv2.imread(pic_file) # 读入图像
>>> im_gray = cv2.cvtColor(im_bgr, cv2.COLOR_BGR2GRAY) # 转灰度
>>> im_gray = cv2.GaussianBlur(im_gray, (3,3), 0) # 滤波降噪
>>> im_edge = cv2.Canny(im_gray, 30, 50) # 边缘检测
>>> cv2.imshow('Go', im_edge) # 显示边缘检测结果
下图是灰度图、滤波降噪后的灰度图和边缘检测的结果。
看起来杂乱无章,但整个棋盘的边缘还是非常清晰完整的。
如果边缘检测效果不佳,可以考虑在边缘检测之前做锐化处理,或者在边缘检测之后做膨胀处理。
3.2 识别并定位棋盘
基于以下约定,我们可以从边缘检测的结果中识别并提取出棋盘的位置信息:
在检测结果中棋盘边缘是清晰完整的
棋盘边缘围成的封闭区域是所有封闭区域中面积最大的
识别棋盘的第一步是使用 OpenCV 的 findContours()函数提取轮廓,该函数返回全部轮廓的列表 contours,以及每个轮廓的属性 hierarchy。
contours 中的每一个轮廓由该轮廓的各个点的坐标来描述,而 hierarchy 则表示每一个轮廓和其他轮廓的相对关系。仅使用轮廓数据列表 contours 就可以找到棋盘。
>>> contours, hierarchy = cv2.findContours(im_edge, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) # 提取轮廓
>>> rect, area = None, 0 # 找到的最大四边形及其面积
>>> for item in contours:
hull = cv2.convexHull(item) # 寻找凸包
epsilon = 0.1 * cv2.arcLength(hull, True) # 忽略弧长10%的点
approx = cv2.approxPolyDP(hull, epsilon, True) # 将凸包拟合为多边形
if len(approx) == 4 and cv2.isContourConvex(approx): # 如果是凸四边形
ps = np.reshape(approx, (4,2))
ps = ps[np.lexsort((ps[:,0],))]
lt, lb = ps[:2][np.lexsort((ps[:2,1],))]
rt, rb = ps[2:][np.lexsort((ps[2:,1],))]
a = cv2.contourArea(approx) # 计算四边形面积
if a > area:
area = a
rect = (lt, lb, rt, rb)
>>> if rect is None:
print('在图像文件中找不到棋盘!')
else:
print('棋盘坐标:')
print('\t左上角:(%d,%d)'%(rect[0][0],rect[0][1]))
print('\t左下角:(%d,%d)'%(rect[1][0],rect[1][1]))
print('\t右上角:(%d,%d)'%(rect[2][0],rect[2][1]))
print('\t右下角:(%d,%d)'%(rect[3][0],rect[3][1]))
棋盘坐标:
左上角:(111,216)
左下角:(47,859)
右上角:(753,204)
右下角:(823,859)
下面的代码将这 4 个点用红色的十字标注在原始的彩色图像上。
>>> im = np.copy(im_bgr)
>>> for p in rect:
im = cv2.line(im, (p[0]-10,p[1]), (p[0]+10,p[1]), (0,0,255), 1)
im = cv2.line(im, (p[0],p[1]-10), (p[0],p[1]+10), (0,0,255), 1)
>>> cv2.imshow('go', im)
用红色十字标注的棋盘四角如下图所示。
很显然,因为拍摄的角度问题,大多数情况下棋盘并不是一个矩形,因此需要对棋盘做透视矫正,使其看起来更像一块真正的棋盘。
3.3 透视矫正
假定透视变换后生成的棋盘大小为 640x640 像素,加上四边各有 10 个像素的空白,图像分辨率为 660x660 像素。
>>> lt, lb, rt, rb = rect
>>> pts1 = np.float32([(10,10), (10,650), (650,10), (650,650)]) # 预期的棋盘四个角的坐标
>>> pts2 = np.float32([lt, lb, rt, rb]) # 当前找到的棋盘四个角的坐标
>>> m = cv2.getPerspectiveTransform(pts2, pts1) # 生成透视矩阵
>>> board_gray = cv2.warpPerspective(im_gray, m, (660, 660)) # 对灰度图执行透视变换
>>> board_bgr = cv2.warpPerspective(im_bgr, m, (660, 660)) # 对彩色图执行透视变换
>>> cv2.imshow('go', board_gray)
下图是经过透视矫正后的棋盘。
现在,我们找到了棋盘的四个角,还需要进一步确定棋盘上每一个交叉格点的坐标,也就是定位棋盘格子。
3.4 定位棋盘格子
透视矫正后的棋盘是边长为 660 像素的正方形、且棋盘格子一定位于棋盘中心,基于这个条件,我们可以简化定位棋盘格子的思路如下:
借助于圆检测,找出棋盘上的每一颗棋子,但要尽量避免找到实际并不存在的棋子
对全部棋子的 x 坐标排序,找出最左侧和最右侧的两列棋子,分别计算其x坐标排序的平均值 x_min 和 x_max
对全部棋子的 y 坐标排序,找出最上方和最下方的两行棋子,分别计算其y坐标排序的平均值 y_min 和 y_max
考察 x 和 y 坐标极值差,最接近 600 者,为棋盘格子的宽度和高度
在分辨率为 660x660 像素的图像上计算出 19x19 的网格四角的坐标
将棋盘映射到 620x620 像素的图像上,网格四角的坐标分别是(22, 22)、 (22, 598)、 (598, 22)和 (598, 598),网格的水平和垂直间距都是 32 像素
>>> circles = cv2.HoughCircles(board_gray, cv2.HOUGH_GRADIENT, 1, 20, param1=90, param2=16, minRadius=10, maxRadius=20) # 圆检测
>>> xs = circles[0,:,0] # 所有棋子的x坐标
>>> ys = circles[0,:,1] # 所有棋子的y坐标
>>> xs.sort()
>>> ys.sort()
>>> k = 1
>>> while xs[k]-xs[:k].mean() < 15:
k += 1
>>> x_min = int(round(xs[:k].mean()))
>>> k = 1
>>> while ys[k]-ys[:k].mean() < 15:
k += 1
>>> y_min = int(round(ys[:k].mean()))
>>> k = -1
>>> while xs[k:].mean() - xs[k-1] < 15:
k -= 1
>>> x_max = int(round(xs[k:].mean()))
>>> k = -1
>>> while ys[k:].mean() - ys[k-1] < 15:
k -= 1
>>> y_max = int(round(ys[k:].mean()))
>>> x_min, x_max, y_min, y_max
(32, 629, 29, 622)
>>> if abs(600-(x_max-x_min)) < abs(600-(y_max-y_min)):
v_min, v_max = x_min, x_max
else:
v_min, v_max = y_min, y_max
>>> v_min, v_max
(32, 629)
>>> lt = (v_min, v_min) # 棋盘网格左上角
>>> lb = (v_min, v_max) # 棋盘网格左下角
>>> rt = (v_max, v_min) # 棋盘网格右上角
>>> rb = (v_max, v_max) # 棋盘网格右下角
>>> pts1 = np.float32([[22, 22], [22, 598], [598, 22], [598, 598]]) # 棋盘四个角点的最终位置
>>> pts2 = np.float32([lt, lb, rt, rb])
>>> m = cv2.getPerspectiveTransform(pts2, pts1)
>>> board_gray = cv2.warpPerspective(board_gray, m, (620, 620))
>>> board_bgr = cv2.warpPerspective(board_bgr, m, (620, 620))
>>> cv2.imshow('go', board_gray)
>>> im = np.copy(board_bgr)
>>> series = np.linspace(22, 598, 19, dtype=np.int)
>>> for i in series:
im = cv2.line(im, (22, i), (598, i), (0,255,0), 1)
im = cv2.line(im, (i, 22), (i, 598), (0,255,0), 1)
>>> cv2.imshow('go', im)
定位棋盘格子之后的效果如下图所示。
确定了棋盘上每一个格子的位置,接下来就是识别无子、黑子、白子三种状态了。
3.5 识别棋子及其颜色
识别棋子之前,再次做圆检测。和上次的圆检测不同,这次要尽可能找到所有的棋子,即使找出来并不存在的棋子也没关系。另一个准备工作是将彩色图像的 BGR 模式转换为 HSV,以便在判断颜色时可以消除明暗、色温等干扰因素。
识别过程就是遍历每一个检测到的圆,在圆心处取 10x10 像素的图像,计算 S 通道和 H 通道的均值,并判断是否存在棋子以及棋子的颜色。代码中的阈值为经验值。识别棋子颜色,亦可考虑使用机器学习的方式实现。
>>> mesh = np.linspace(22, 598, 19, dtype=np.int)
>>> rows, cols = np.meshgrid(mesh, mesh)
>>> circles = cv2.HoughCircles(board_gray, cv2.HOUGH_GRADIENT, 1, 20, param1=40, param2=10, minRadius=12, maxRadius=18) # 再做一次圆检测
>>> circles = np.uint32(np.around(circles[0]))
>>> phase = np.zeros_like(rows, dtype=np.uint8)
>>> im_hsv = cv2.cvtColor(board_bgr, cv2.COLOR_BGR2HSV_FULL)
>>> for circle in circles:
row = int(round((circle[1]-22)/32))
col = int(round((circle[0]-22)/32))
hsv_ = im_hsv[cols[row,col]-5:cols[row,col]+5, rows[row,col]-5:rows[row,col]+5]
s = np.mean(hsv_[:,:,1])
v = np.mean(hsv_[:,:,2])
if 0 < v < 115:
phase[row,col] = 1 # 黑棋
elif 0 < s < 50 and 114 < v < 256:
phase[row,col] = 2 # 白棋
>>> phase
array([[1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 1],
[0, 1, 1, 1, 2, 2, 2, 1, 2, 1, 1, 2, 0, 2, 1, 2, 1, 1, 1],
[0, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 1, 1, 2, 1, 0],
[1, 1, 1, 2, 2, 0, 2, 0, 0, 2, 2, 1, 1, 1, 1, 1, 0, 0, 0],
[1, 2, 2, 1, 1, 2, 2, 2, 0, 0, 2, 2, 1, 0, 1, 0, 0, 0, 0],
[2, 2, 1, 1, 1, 2, 1, 1, 2, 2, 1, 1, 0, 1, 0, 1, 1, 0, 0],
[0, 2, 2, 1, 0, 1, 1, 0, 1, 2, 2, 1, 0, 0, 1, 1, 1, 1, 0],
[0, 0, 2, 1, 1, 2, 1, 1, 1, 2, 1, 1, 0, 1, 2, 2, 1, 0, 1],
[0, 2, 1, 1, 1, 2, 2, 1, 1, 1, 2, 1, 0, 1, 0, 2, 2, 0, 0],
[0, 0, 2, 2, 1, 1, 2, 2, 2, 2, 2, 1, 1, 2, 2, 2, 0, 0, 0],
[0, 2, 2, 1, 1, 2, 2, 0, 2, 2, 2, 2, 1, 1, 2, 1, 0, 2, 1],
[2, 2, 2, 1, 1, 1, 2, 2, 1, 1, 2, 2, 2, 2, 1, 1, 1, 0, 1],
[1, 2, 1, 1, 2, 2, 2, 1, 0, 1, 2, 2, 1, 2, 2, 2, 1, 1, 0],
[1, 1, 0, 1, 1, 1, 2, 1, 1, 1, 1, 2, 1, 2, 2, 2, 2, 2, 0],
[0, 0, 1, 2, 1, 2, 2, 2, 1, 0, 1, 2, 1, 2, 1, 1, 1, 2, 1],
[1, 1, 2, 2, 2, 2, 0, 2, 2, 0, 2, 2, 1, 2, 2, 2, 1, 2, 1],
[1, 1, 2, 0, 0, 1, 2, 2, 1, 1, 2, 1, 1, 2, 1, 1, 1, 1, 0],
[1, 2, 2, 0, 0, 2, 2, 0, 2, 1, 1, 2, 1, 1, 1, 0, 0, 1, 1],
[2, 2, 2, 0, 0, 0, 0, 2, 2, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0]],
dtype=uint8)
源码文件
4.1 统计棋子和围空数量的脚本文件
stats.py
# -*- coding: utf-8 -*-
"""
根据围棋局面,计算对局结果
使用NumPy二维ubyte数组存储局面,约定:
0 - 空
1 - 黑子
2 - 白子
3 - 黑空
4 - 白空
5 - 黑子统计标识
6 - 白子统计标识
7 - 黑残子
8 - 白残子
9 - 单官/公气/统计标识
"""
import numpy as np
import os
os.system('')
def show_phase(phase):
"""显示局面"""
for i in range(19):
for j in range(19):
if phase[i,j] == 1:
chessman = chr(0x25cf)
elif phase[i,j] == 2:
chessman = chr(0x25cb)
elif phase[i,j] == 9:
chessman = chr(0x2606)
else:
if i == 0:
if j == 0:
chessman = '%s '%chr(0x250c)
elif j == 18:
chessman = '%s '%chr(0x2510)
else:
chessman = '%s '%chr(0x252c)
elif i == 18:
if j == 0:
chessman = '%s '%chr(0x2514)
elif j == 18:
chessman = '%s '%chr(0x2518)
else:
chessman = '%s '%chr(0x2534)
elif j == 0:
chessman = '%s '%chr(0x251c)
elif j == 18:
chessman = '%s '%chr(0x2524)
else:
chessman = '%s '%chr(0x253c)
print('\033[0;30;43m' + chessman + '\033[0m', end='')
print()
def find_blank(phase, cell):
"""找出包含cell的成片的空格"""
def _find_blank(phase, result, cell):
i, j = cell
phase[i,j] = 9
result['cross'].add(cell)
if i-1 > -1:
if phase[i-1,j] == 0:
_find_blank(phase, result, (i-1,j))
elif phase[i-1,j] == 1:
result['b_around'].add((i-1,j))
elif phase[i-1,j] == 2:
result['w_around'].add((i-1,j))
if i+1 < 19:
if phase[i+1,j] == 0:
_find_blank(phase, result, (i+1,j))
elif phase[i+1,j] == 1:
result['b_around'].add((i+1,j))
elif phase[i+1,j] == 2:
result['w_around'].add((i+1,j))
if j-1 > -1:
if phase[i,j-1] == 0:
_find_blank(phase, result, (i,j-1))
elif phase[i,j-1] == 1:
result['b_around'].add((i,j-1))
elif phase[i,j-1] == 2:
result['w_around'].add((i,j-1))
if j+1 < 19:
if phase[i,j+1] == 0:
_find_blank(phase, result, (i,j+1))
elif phase[i,j+1] == 1:
result['b_around'].add((i,j+1))
elif phase[i,j+1] == 2:
result['w_around'].add((i,j+1))
result = {'cross':set(), 'b_around':set(), 'w_around':set()}
_find_blank(phase, result, cell)
return result
def find_blanks(phase):
"""找出所有成片的空格"""
blanks = list()
while True:
cells = np.where(phase==0)
if cells[0].size == 0:
break
blanks.append(find_blank(phase, (cells[0][0], cells[1][0])))
return blanks
def stats(phase):
"""统计结果"""
temp = np.copy(phase)
for item in find_blanks(np.copy(phase)):
if len(item['w_around']) == 0:
v = 3 # 黑空
elif len(item['b_around']) == 0:
v = 4 # 白空
else:
v = 9 # 单官或公气
for i, j in item['cross']:
temp[i, j] = v
black = temp[temp==1].size + temp[temp==3].size
white = temp[temp==2].size + temp[temp==4].size
common = temp[temp==9].size
return black, white, common
if __name__ == '__main__':
phase = np.array([
[0,0,2,1,1,0,1,1,1,2,0,2,0,2,1,0,1,0,0],
[0,0,2,1,0,1,1,1,2,0,2,0,2,2,1,1,1,0,0],
[0,0,2,1,1,0,0,1,2,2,0,2,0,2,1,0,1,0,0],
[0,2,1,0,1,1,0,1,2,0,2,2,2,0,2,1,0,1,0],
[0,2,1,1,0,1,1,2,2,2,2,0,0,2,2,1,0,1,0],
[0,0,2,1,1,1,1,2,0,2,0,2,0,0,2,1,0,0,0],
[0,0,2,2,2,2,1,2,2,0,0,0,0,0,2,1,0,0,0],
[2,2,2,0,0,0,2,1,1,2,0,2,0,0,2,1,0,0,0],
[1,1,2,0,0,0,2,2,1,2,0,0,0,0,2,1,0,0,0],
[1,0,1,2,0,2,1,1,1,1,2,2,2,0,2,1,1,1,1],
[0,1,1,2,0,2,1,0,0,0,1,2,0,2,2,1,0,0,1],
[1,1,2,2,2,2,2,1,0,0,1,2,2,0,2,1,0,0,0],
[2,2,0,2,2,0,2,1,0,0,1,2,0,2,2,2,1,0,0],
[0,2,0,0,0,0,2,1,0,1,1,2,2,0,2,1,0,0,0],
[0,2,0,0,0,2,1,0,0,1,0,1,1,2,2,1,0,0,0],
[0,0,2,0,2,2,1,1,1,1,0,1,0,1,1,0,0,0,0],
[0,2,2,0,2,1,0,0,0,0,1,0,0,0,0,1,1,0,0],
[0,0,2,0,2,1,0,1,1,0,0,1,0,1,0,1,0,0,0],
[0,0,0,2,1,1,0,0,0,0,0,0,0,0,1,0,0,0,0]
], dtype=np.ubyte)
show_phase(phase)
black, white, common = stats(phase)
print('--------------------------------------')
print('黑方:%d,白方:%d,公气:%d'%(black, white, common))
4.2 视觉识别的脚本文件
go.py
# -*- coding: utf-8 -*-
"""
识别图像中的围棋局面
"""
import cv2
import numpy as np
from stats import show_phase, stats
class GoPhase:
"""从图片中识别围棋局面"""
def __init__(self, pic_file, offset=3.75):
"""构造函数,读取图像文件,预处理"""
self.offset = offset # 黑方贴七目半
self.im_bgr = cv2.imread(pic_file) # 原始的彩色图像文件,BGR模式
self.im_gray = cv2.cvtColor(self.im_bgr, cv2.COLOR_BGR2GRAY) # 转灰度图像
self.im_gray = cv2.GaussianBlur(self.im_gray, (3,3), 0) # 灰度图像滤波降噪
self.im_edge = cv2.Canny(self.im_gray, 30, 50) # 边缘检测获得边缘图像
self.board_gray = None # 棋盘灰度图
self.board_bgr = None # 棋盘彩色图
self.rect = None # 棋盘四个角的坐标,顺序为lt/lb/rt/rb
self.phase = None # 用以表示围棋局面的二维数组
self.result = None # 对弈结果
self._find_chessboard() # 找到棋盘
self._location_grid() # 定位棋盘格子
self._identify_chessman() # 识别棋子
self._stats() # 统计黑白双方棋子和围空
def _find_chessboard(self):
"""找到棋盘"""
contours, hierarchy = cv2.findContours(self.im_edge, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) # 提取轮廓
area = 0 # 找到的最大四边形及其面积
for item in contours:
hull = cv2.convexHull(item) # 寻找凸包
epsilon = 0.1 * cv2.arcLength(hull, True) # 忽略弧长10%的点
approx = cv2.approxPolyDP(hull, epsilon, True) # 将凸包拟合为多边形
if len(approx) == 4 and cv2.isContourConvex(approx): # 如果是凸四边形
ps = np.reshape(approx, (4,2)) # 四个角的坐标
ps = ps[np.lexsort((ps[:,0],))] # 排序区分左右
lt, lb = ps[:2][np.lexsort((ps[:2,1],))] # 排序区分上下
rt, rb = ps[2:][np.lexsort((ps[2:,1],))] # 排序区分上下
a = cv2.contourArea(approx)
if a > area:
area = a
self.rect = (lt, lb, rt, rb)
if not self.rect is None:
pts1 = np.float32([(10,10), (10,650), (650,10), (650,650)]) # 预期的棋盘四个角的坐标
pts2 = np.float32(self.rect) # 当前找到的棋盘四个角的坐标
m = cv2.getPerspectiveTransform(pts2, pts1) # 生成透视矩阵
self.board_gray = cv2.warpPerspective(self.im_gray, m, (660, 660)) # 执行透视变换
self.board_bgr = cv2.warpPerspective(self.im_bgr, m, (660, 660)) # 执行透视变换
def _location_grid(self):
"""定位棋盘格子"""
if self.board_gray is None:
return
circles = cv2.HoughCircles(self.board_gray, cv2.HOUGH_GRADIENT, 1, 20, param1=90, param2=16, minRadius=10, maxRadius=20) # 圆检测
xs, ys = circles[0,:,0], circles[0,:,1] # 所有棋子的x坐标和y坐标
xs.sort()
ys.sort()
k = 1
while xs[k]-xs[:k].mean() < 15:
k += 1
x_min = int(round(xs[:k].mean()))
k = 1
while ys[k]-ys[:k].mean() < 15:
k += 1
y_min = int(round(ys[:k].mean()))
k = -1
while xs[k:].mean() - xs[k-1] < 15:
k -= 1
x_max = int(round(xs[k:].mean()))
k = -1
while ys[k:].mean() - ys[k-1] < 15:
k -= 1
y_max = int(round(ys[k:].mean()))
if abs(600-(x_max-x_min)) < abs(600-(y_max-y_min)):
v_min, v_max = x_min, x_max
else:
v_min, v_max = y_min, y_max
pts1 = np.float32([[22, 22], [22, 598], [598, 22], [598, 598]]) # 棋盘四个角点的最终位置
pts2 = np.float32([(v_min, v_min), (v_min, v_max), (v_max, v_min), (v_max, v_max)])
m = cv2.getPerspectiveTransform(pts2, pts1)
self.board_gray = cv2.warpPerspective(self.board_gray, m, (620, 620))
self.board_bgr = cv2.warpPerspective(self.board_bgr, m, (620, 620))
def _identify_chessman(self):
"""识别棋子"""
if self.board_gray is None:
return
mesh = np.linspace(22, 598, 19, dtype=np.int)
rows, cols = np.meshgrid(mesh, mesh)
circles = cv2.HoughCircles(self.board_gray, cv2.HOUGH_GRADIENT, 1, 20, param1=40, param2=10, minRadius=12, maxRadius=18)
circles = np.uint32(np.around(circles[0]))
self.phase = np.zeros_like(rows, dtype=np.uint8)
im_hsv = cv2.cvtColor(self.board_bgr, cv2.COLOR_BGR2HSV_FULL)
for circle in circles:
row = int(round((circle[1]-22)/32))
col = int(round((circle[0]-22)/32))
hsv_ = im_hsv[cols[row,col]-5:cols[row,col]+5, rows[row,col]-5:rows[row,col]+5]
s = np.mean(hsv_[:,:,1])
v = np.mean(hsv_[:,:,2])
if 0 < v < 115:
self.phase[row,col] = 1 # 黑棋
elif 0 < s < 50 and 114 < v < 256:
self.phase[row,col] = 2 # 白棋
def _stats(self):
"""统计黑白双方棋子和围空"""
self.result = stats(self.phase)
def show_image(self, name='gray', win="GoPhase"):
"""显示图像"""
if name == 'bgr':
im = self.board_bgr
elif name == 'gray':
im = self.board_gray
else:
im = self.im_bgr
if im is None:
print('识别失败,无图像可供显示')
else:
cv2.imshow(win, im)
cv2.waitKey(0)
cv2.destroyAllWindows()
def show_phase(self):
"""显示局面"""
if self.phase is None:
print('识别失败,无围棋局面可供显示')
else:
show_phase(self.phase)
def show_result(self):
"""显示结果"""
if self.result is None:
print('识别失败,无对弈结果可供显示')
else:
black, white, common = self.result
B = black+common/2-self.offset
W = white+common/2+self.offset
result = '黑胜' if B > W else '白胜'
print('黑方:%0.1f,白方:%0.1f,%s'%(B, W, result))
if __name__ == '__main__':
go = GoPhase('res/pic_0.jpg')
go.show_image('origin')
go.show_image('gray')
go.show_phase()
go.show_result()
更多精彩推荐 ☞程序员为教师妻子开发专属应用;2020 最佳开源项目出炉;中国构建全星地量子通信网|开发者周刊
点分享 点收藏 点点赞 点在看
关注公众号:拾黑(shiheibook)了解更多
[广告]赞助链接:
四季很好,只要有你,文娱排行榜:https://www.yaopaiming.com/
让资讯触达的更精准有趣:https://www.0xu.cn/
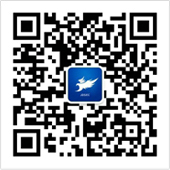
随时掌握互联网精彩
- 1 习主席致敬老战士的动人瞬间 7904787
- 2 中美经贸高层会谈在瑞士举行 7809580
- 3 本科生发14篇SCI 副院长父亲被免职 7713738
- 4 为中国经济注入更多确定性 7615999
- 5 普京与巴西总统会谈 举着耳机不说话 7522890
- 6 上门做饭月薪近2万被质疑 女子回应 7423847
- 7 网警查处一起法律服务公司侵公案 7330530
- 8 印度称不会升级冲突 7231445
- 9 男子地铁上做怪异行为致3人恐慌受伤 7139765
- 10 关税乱拳打乱不了我们的发展节奏 7048073